Creating a Sample Editor
This procedure creates a sample editor that uses Sabre Red 360 classes. The sample editor uses these classes because Sabre Red 360 classes include most of the behaviors that developers and end-users require. In addition, the procedure includes sample code that an editor uses. An example of the plugin.xml file that this procedure builds is provided.
Before you create the sample editor, create a new plug-in project or use an existing project.
-
In Eclipse Package Explorer view, open your project, and then open the Extensions tab.
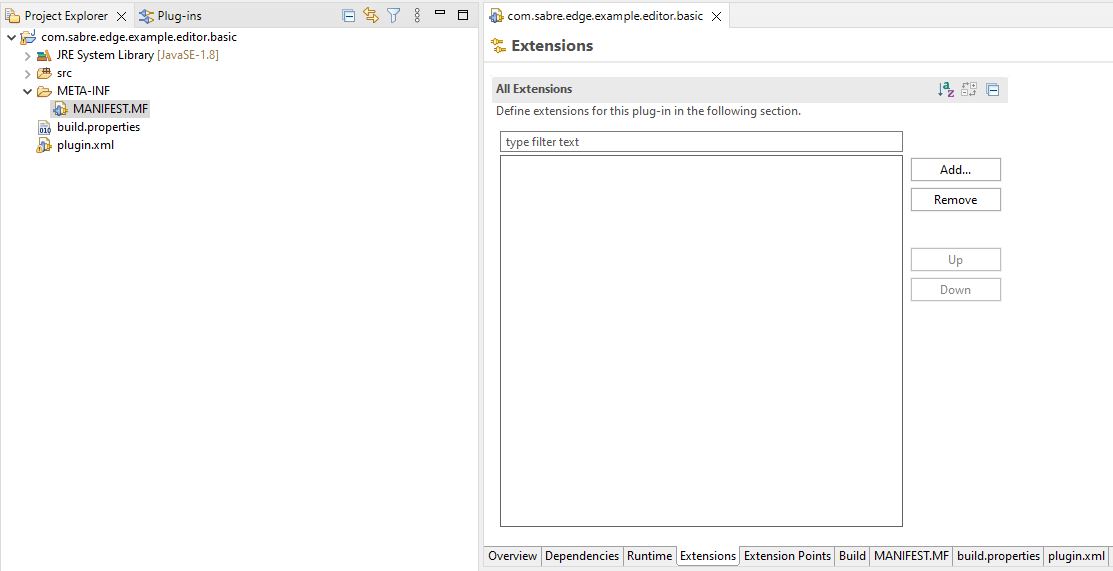
-
Click Add to open the New Extension dialog.
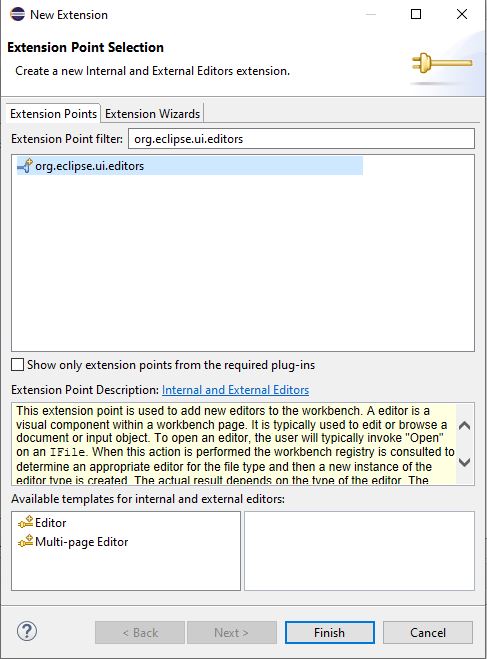
-
Remove the selection from Show Only Extension Points from the Required Plug-ins.
-
In the Extension Point Filter field, type org.eclipse.ui.editors.
You can type either the complete name or * followed by the partial name to display a list of names that contain the characters you enter. Select org.eclipse.ui.editors when it appears in the list.
-
Click Finish.
-
If Eclipse displays the following New Plug-in Dependency dialog, click Yes.
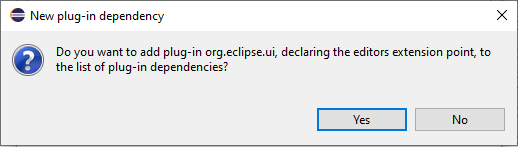
Adding Minimal Required Dependencies for Sabre Red 360
If you do not have a project that uses required dependencies for Sabre Red 360, you must add them. If you have logic in your project, these dependencies are already present.
-
Open the Dependencies tab.
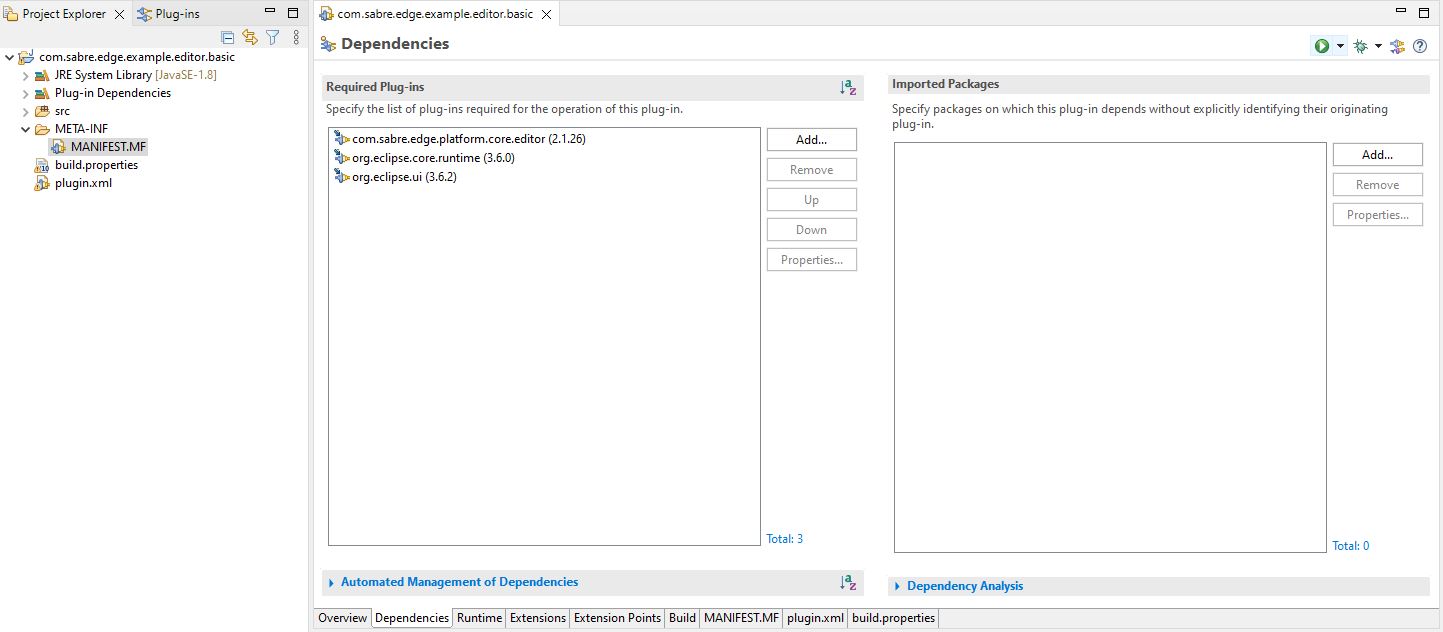
-
Add the following required dependencies.
-
org.eclipse.core.runtime
-
com.sabre.edge.platform.core.editor
-
Eclipse automatically adds the org.eclipse.ui dependency because the plug-in project was set up to contribute to the UI.
-
Open the Extensions tab. Eclipse adds the org.eclipse.ui.editors extension point and a new editor element. If Eclipse does not add the editor element, right-click org.eclipse.ui.editors, and then choose New > editor.
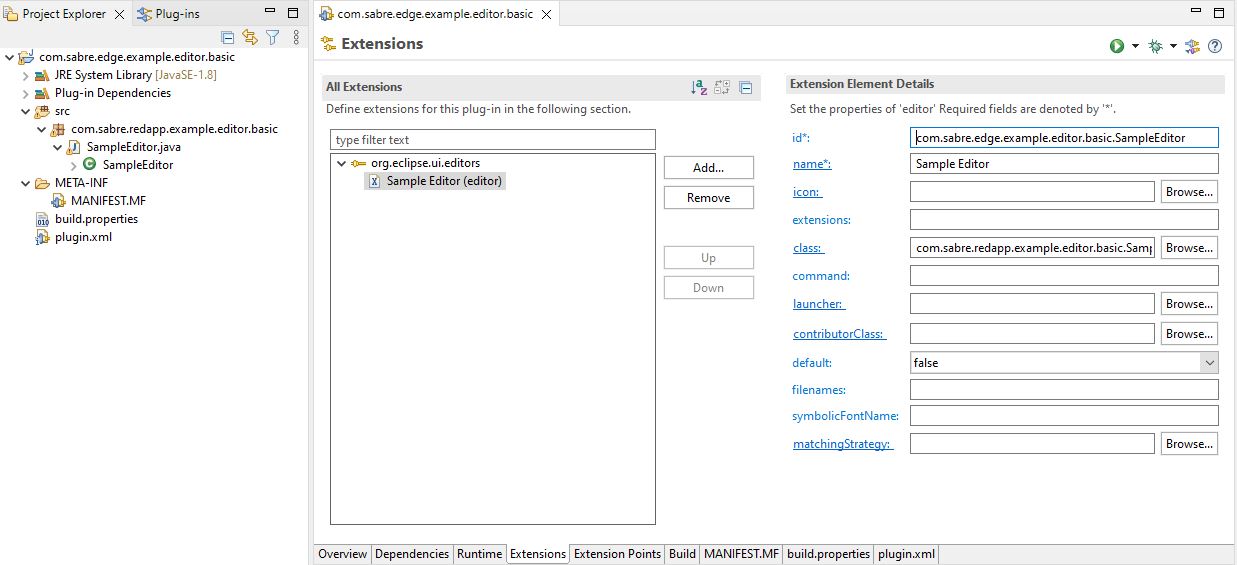
-
In Extension Element Details, add properties to the editor element in the following fields:
id |
The value in id does not match anything now, but this value is used later. |
name |
This name appears on the Extensions tab in Eclipse to make it easy to identify the editor. |
icon |
This is the icon to associate with the editor. The icon appears on the tab next to the label. |
-
In Extension Element Details, click the class link. The New Java Class dialog is displayed.
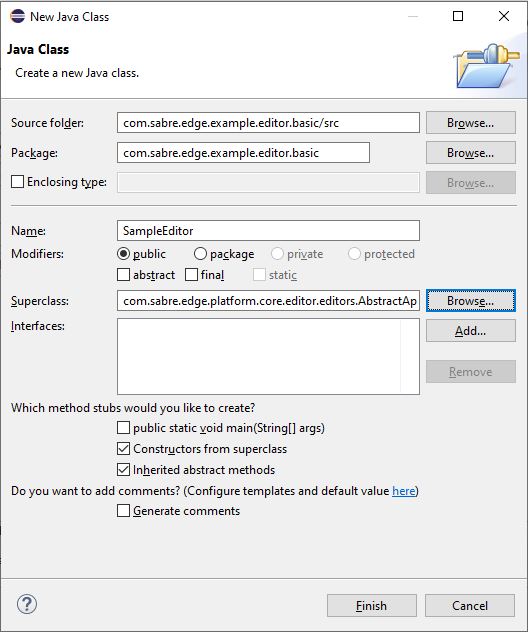
When you create an editor, always implement your own class as a subclass of the Sabre Red 360 AbstractAppEditor class.
-
If the Package field is not populated, type the package name. Sample package: com.sabre.redapp.example.editor.basic
-
In the Name field, type the same value that you entered in the id field for the org.eclipse.ui.editors extension. Your editor class must extend com.sabre.edge.platform.core.editor.editors.AbstractAppEditor. Sample value: SampleEditor
-
In the Superclass field, type com.sabre.edge.platform.core.editor.editors.AbstractAppEditor.
-
Select the following check boxes:
-
Constructors from superclass
-
Inherited abstract methods
-
-
Click Finish.
You are done adding a new extension with dependencies and properties.
Sample Java code for the extended AbstractAppEditor is shown below. This code sample creates a label object named Sample Editor. The code has the class name that you added to extend AbstractAppEditor, and it describes the label with your text.
package com.sabre.redapp.example.editor.basic;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Label;
import com.sabre.edge.platform.core.editor.editors.AbstractAppEditor;
public class SampleEditor extends AbstractAppEditor {
public SampleEditor() {
super();
}
public void createPartControl(Composite parent) {
progressAnimation.init(parent);
Label label = new Label(parent, SWT.NONE);
label.setText("Sample Editor");
parent.setLayout(new FillLayout());
}
}
-
Add the createPartControl() method manually now. This method allows you to create user interface elements, such as buttons or labels.
Adding the org.eclipse.ui.elementFactories Extension for the Element Factory
-
On the Extensions tab, click Add to display the New Extension dialog.
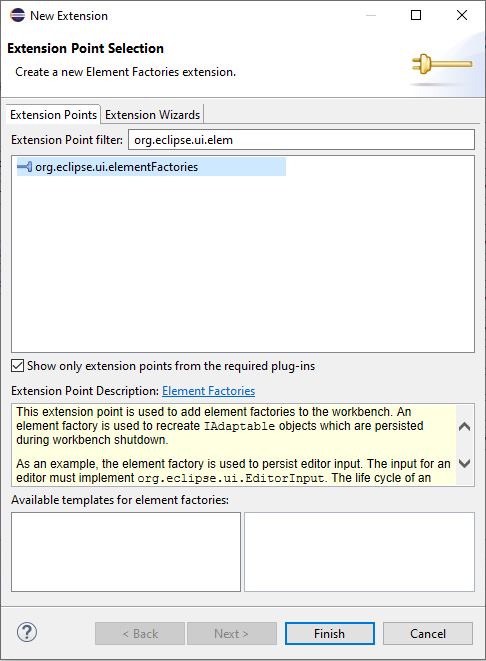
-
Select Show Only Extension Points from the Required Plug-ins.
-
In the Extension Point Filter field, type org.eclipse.ui.elementFactories.
-
Click Finish.
-
Eclipse adds the org.eclipse.ui.elementFactories extension and the factory element to the Extensions tab. If Eclipse does not add the factory element, right-click org.eclipse.ui.elementFactories, and then choose New > factory.
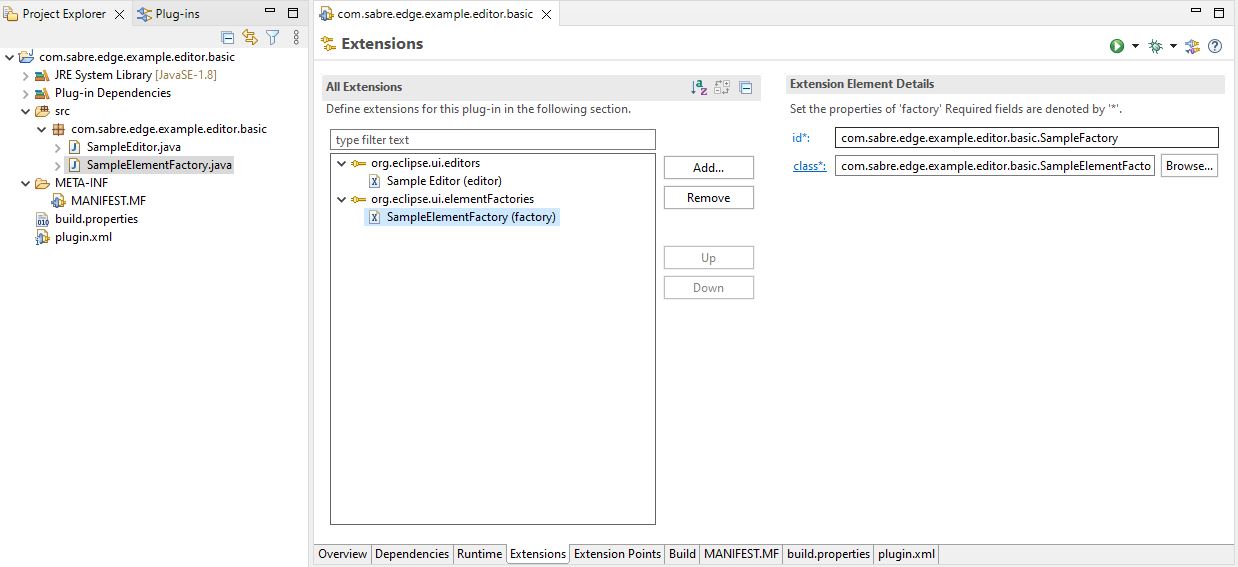
-
Add the id property to factory.
Type the fully-qualified name of your package, followed by a name for the type. In the sample editor, this value is com.sabre.redapp.example.editor.basic.SampleElementFactory .
-
Click the class link to display the New Java Class dialog.
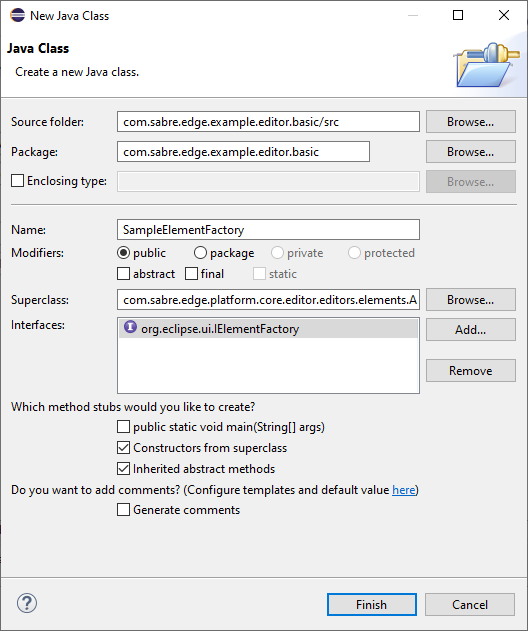
On this dialog, you will extend the com.sabre.edge.platform.core.editor.editors.elements.AbstractElementFactory class.
-
If the Package field is not populated, type the package name. Sample package: com.sabre.redapp.example.editor.basic
-
In the Name field, type the name of your class. Your class must extend com.sabre.edge.platform.core.editor.editors.elements.AbstractElementFactory. Sample name: SampleElementFactory
-
In the Superclass field, type the following:
com.sabre.edge.platform.core.editor.editors.elements.AbstractElementFactory
Your class must implement the getEditorInput() method, and return the appropriate instance of the class to extend the following:
com.sabre.edge.platform.core.editor.editors.inputs.AbstractEditorInput
In the sample editor, your class is SampleElementFactory .
-
In the Interfaces field, add org.eclipse.ui.IElementFactory.
-
Select the following check boxes:
-
Constructors from superclass
-
Inherited abstract methods
-
-
Click Finish.
Sample Java code for the SampleEditorInput class is shown below. In this code sample, AbstractEditorInput implements getFactoryId() and returns the same value for ID that you defined in org.eclipse.ui.elementFactories .
package com.sabre.redapp.example.editor.basic;
import com.sabre.edge.platform.core.editor.editors.inputs.AbstractEditorInput;
public class SampleEditorInput extends AbstractEditorInput {
@Override
public String getFactoryId() {
return SampleElementFactory.ID;
}
}
Sample Java code for getEditorInput() is shown below. In this code sample, getEditorInput() returns the SampleEditorInput to com.sabre.edge.platform.core.editor.editors.inputs.AbstractEditorInput.
package com.sabre.redapp.example.editor.basic;
import org.eclipse.ui.IElementFactory;
import com.sabre.edge.platform.core.editor.editors.elements.AbstractElementFactory;
import com.sabre.edge.platform.core.editor.editors.inputs.AbstractEditorInput;
public class SampleElementFactory extends AbstractElementFactory implements
IElementFactory {
public static final String ID = "com.sabre.redapp.example.editor.basic.SampleElementFactory";
@Override
public AbstractEditorInput getEditorInput() {
return new SampleEditorInput();
}
}
Adding the com.sabre.edge.platform.core.editor.editorApp Extension
The extension com.sabre.edge.platform.core.editor.editorApp is required for any editor you create for Sabre Red 360.
-
On the Extensions tab, click Add to display the New Extension dialog.
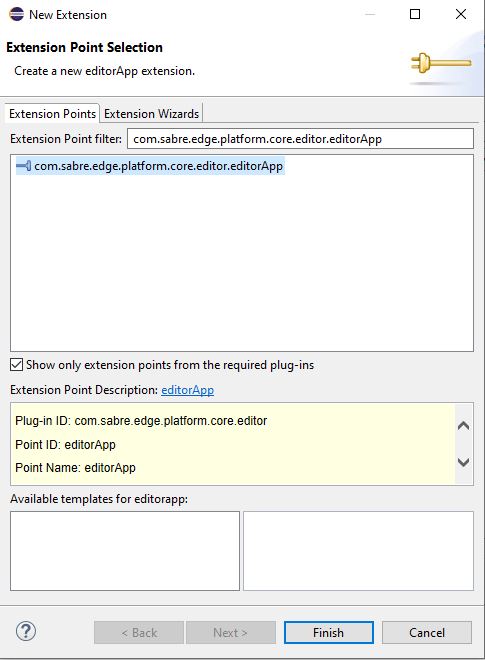
-
Select Show Only Extension Points from the Required Plug-ins.
-
In the Extension Point Filter field, type com.sabre.edge.platform.core.editor.editorApp.
-
Click Finish.
-
Eclipse adds the com.sabre.edge.platform.core.editor.editorApp extension point and a new editorApp element on the Extensions tab. If Eclipse does not add editorApp, right-click com.sabre.edge.platform.core.editor.editorApp. Choose New > editorApp from the menu.
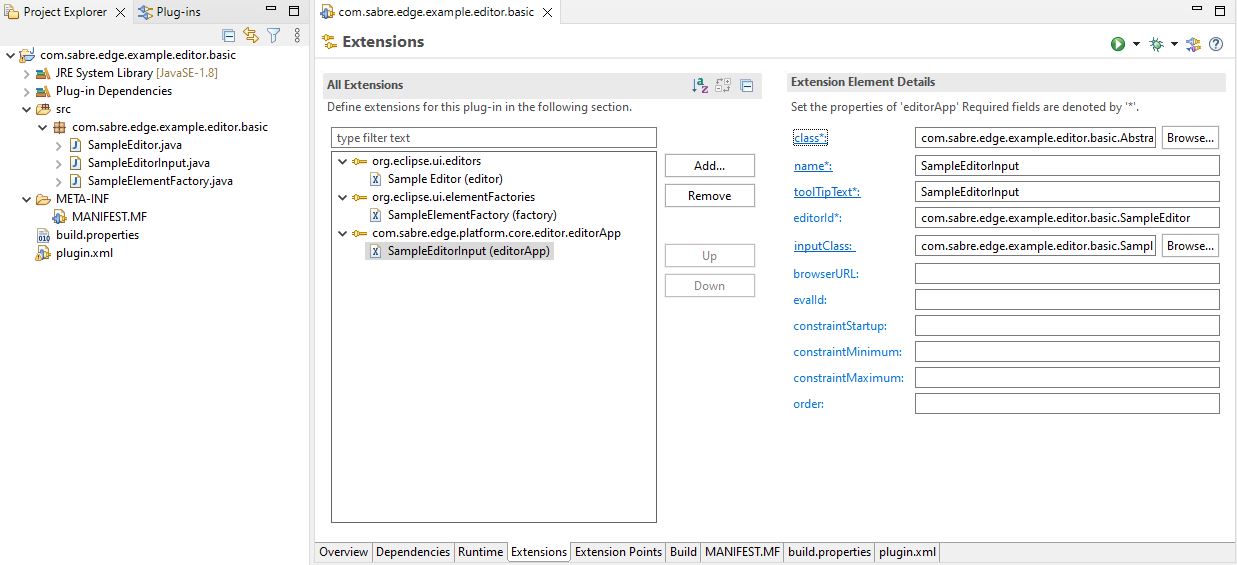
-
Add properties to the editorApp element in the following fields:
class |
The value that you type must match the class that you defined in org.eclipse.ui.editors. |
inputClass |
Type the class that you used in getEditorInput() to extend AbstractEditorInput. |
name |
Type the text for the label that you want to display on the tab. The tab for the editor appears on the GUI. |
toolTipText |
Type the text that end-users see when their mouse hovers over the label on the tab. |
editorId |
This value must match the id that you defined for org.eclipse.ui.editors. This is the first extension that you added. |
-
(Optional) Save MANIFEST.MF.
You are done creating the classes and extensions that you need for an editor.
Next, you must add commands with handlers. Afterwards, you will bind these commands and handlers to the editor.
Adding the org.eclipse.ui.commands Extension
-
On the Extensions tab, click Add to display the New Extension dialog.
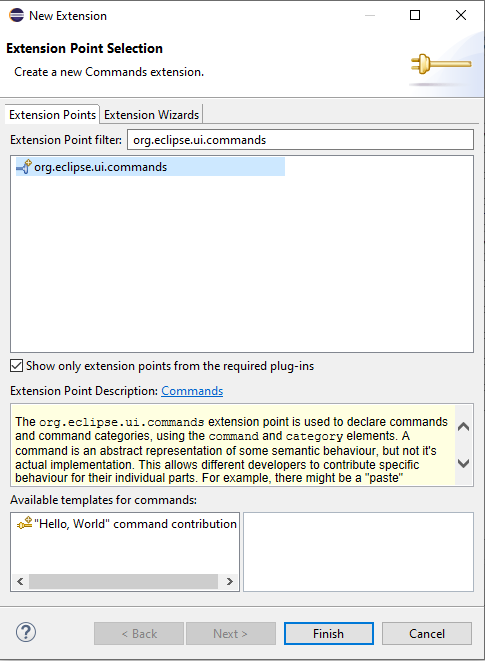
-
Remove the selection from Show only extension points from the required plug-ins.
-
In the Extension Point Filter field, type org.eclipse.ui.commands.
-
Click Finish.
-
If Eclipse displays the New Plug-in Dependency dialog, click Yes.
Eclipse adds the org.eclipse.ui.commands extension point to the Extensions tab. Next, add a new command
for the extension.
-
Right-click org.eclipse.ui.commands, and then choose New > command.
-
Add properties to the command element in the following fields:
id |
This is the command identifier. The value is used throughout the Eclipse application. |
name |
This name appears on the Extensions tab in Eclipse to make it easy to identify the command name. |
Informing the Handler Which Editor to Open with a Command Parameter
-
Add a new parameter to the command org.eclipse.ui.commands. On the Extensions tab, right-click Sample Command, and then choose New > commandParameter. (SampleCommand is the command parameter.)
-
Add properties to the Sample Command element in the following fields:
id |
This is the parameter identifier. |
name |
This is the definition of the parameter that is added to the menuContribution element in org.eclipse.ui.menus. This name does not appear on the GUI. |
Creating a New Handler to Implement the Command
-
On the Extensions tab, click Add. You will add a handlers extension on the New Extension dialog.
-
Remove the selection from Show only extension points from the required plug-ins. In the Extension Point Filter field on the New Extensions screen, type org.eclipse.ui.handlers. Click Finish. If Eclipse displays the New Plug-in Dependency dialog, click Yes. Eclipse adds the org.eclipse.ui.handlers extension point and a new handler element to the Extensions tab. If Eclipse does not add the handler element, right-click org.eclipse.ui.handlers, and then choose New > handler. Add properties to the handler element in the following fields.
commandId |
This must be the identifier of the new command that you selected when you added the org.eclipse.ui.commands extension. |
class |
Instead of creating a new handler class, select the Sabre Red 360 handler class. Click Browse next to the class field. |
-
Save MANIFEST.MF.
View the sample plugin.xml file for this sample editor.