Creating a Dialog
The step-by-step instructions show how to create a dialog using pre-defined methods and classes. Complete the optional sections for the features that you want.
Before you begin, complete the following:
-
Create a new plug-in project or use an existing plug-in project.
-
Create a generic handler. The handler triggers the dialog.
When you are done creating a dialog, it is optional to add a menu contribution to open the dialog.
-
Open your project in the Eclipse Package Explorer view.
-
On the Dependencies tab, add the following required dependencies:
-
org.eclipse.ui
-
com.sabre.edge.platform.core.ui
-
Adding the MySabreTrayDialog Class
-
In Package Explorer, right-click your project name. From the context menu, choose New > Class.
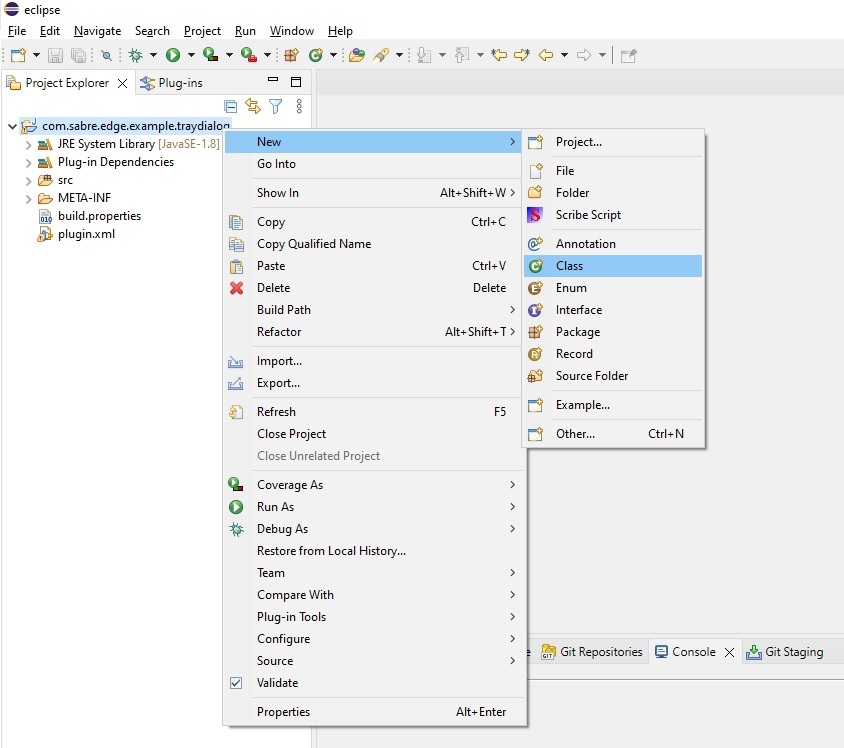
On the New Java Class dialog, you will extend the com.sabre.edge.platform.core.ui.dialogs.MySabreTrayDialog class for the dialog. Your extended class will contain the content and buttons for your dialog.
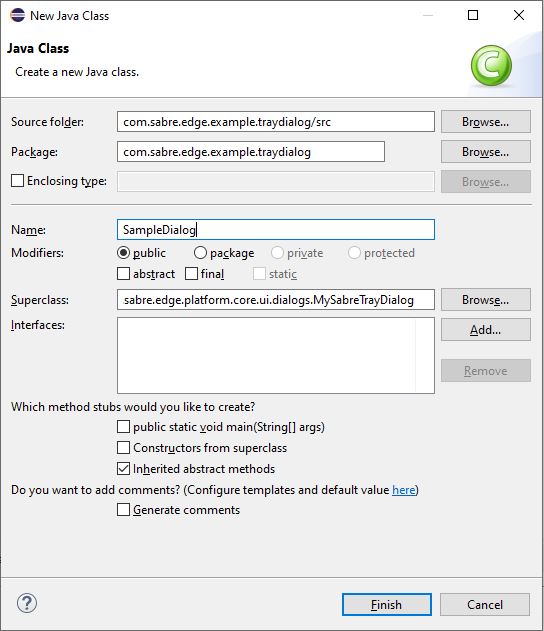
-
If the package field is empty, type the name of your package. Sample package: com.sabre.redapp.example.traydialog
-
In the Name field, type a name for your class. Your class must extend the com.sabre.edge.platform.core.ui.dialogs.MySabreTrayDialog.Sample class name: SampleDialog
-
In Superclass, type com.sabre.edge.platform.core.ui.dialogs.MySabreTrayDialog.
-
Select the following check boxes:
-
Constructors from superclass
-
Inherited abstract methods
-
-
Click Finish.
Creating the Content for the Handler
-
In a Java editor, add the following code in the executing method of the handler class or in the selection listener that will display the dialog. Eclipse does not generate this code.
After you add the following code to your handler or listener, your dialog should appear after the handler or listener is invoked. It should look similar to the figure that follows this sample.
Shell shell =
PlatformUI.getWorkbench().getDisplay().getActiveShell();
Window dialog = new SampleDialog(shell);
int status = dialog.open();
if (status == Dialog.OK)
{
/* Add code for handling the dialog status, if needed */
}
Basic Dialog with the Default OK and Cancel Buttons
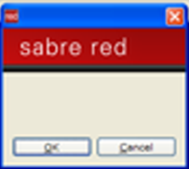
Customizing the Title on the Dialog
-
Add the following code inside the dialog class that you created in a previous step. The super.configureShell() method calls an implementation of configureShell() from the parent class.
/**
* {@inheritDoc}
*/
@Override
protected void configureShell(Shell shell)
{
super.configureShell(shell); /* This calls the configureShell method from the parent class*/
shell.setText("Sample Tray Dialog");
}
Creating Content for the Dialog
-
Overwrite the createDialogArea(Composite parent) method. The super.createDialogArea() method uses pre-defined settings, such as layout and margins. Add the following code in order to create the layout of the dialog inside of the createDialogArea() method.
/**
* {@inheritDoc}
*/
@Override
protected Control createDialogArea(Composite parent)
{
Composite area = (Composite)
super.createDialogArea(parent);
createControl(area);
return area;
}
-
Add code that creates the content of the dialog inside the createControl() method. The following sample shows how the code may look.
Sample Java Code to Create Dialog Content in createControl()
private void createControl(Composite parent) {
Composite composite = new Composite(parent, SWT.NONE);
GridLayout gridLayout = new GridLayout(2, false);
gridLayout.marginWidth = 15;
gridLayout.marginHeight = 10;
gridLayout.horizontalSpacing = 15;
gridLayout.verticalSpacing = 10;
composite.setLayout(gridLayout);
Label image = new Label(composite, SWT.NONE);
image.setImage(Display.getCurrent().getSystemImage(SWT.ICON_QUESTION));
Composite box = createBox(composite, 6);
new Label(box, SWT.NONE).setText("Put your comments below (max 50 characters).");
createText(box);
}
private static Composite createBox(Composite composite, int span)
{
Composite box = new Composite(composite, SWT.NONE);
GridData gridData = new GridData(GridData.FILL_BOTH);
gridData.verticalSpan = span;
box.setLayoutData(gridData);
box.setLayout(new GridLayout());
return box;
}
private void createText(Composite composite)
{
Text text =
new Text(composite, SWT.BORDER SWT.MULTI SWT.WRAP SWT.LEFT SWT.V_SCROLL);
text.setTextLimit(50);
GridData gd = new GridData(GridData.FILL_BOTH);
gd.heightHint = 48;
text.setLayoutData(gd);
}
After you add the code, your dialog should be similar to the following figure.
Dialog with Content in the Form of a Label and Text Box
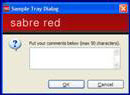
(Optional) Modifying the Default OK and Cancel Button Labels
-
Add the following code to modify the default OK and Cancel button labels.
To obtain a reference to a button, use the getButton() method and pass the identifier of the button.
Eclipse assigns default labels to the OK and Cancel buttons as follows:
IDialogConstants.OK_ID
IDialogConstants.CANCEL.ID
Add setText and pass a value to overwrite the default labels. In the example below, the new values for the buttons are Send
and Skip
.
/**
* {@inheritDoc}
*/
@Override
public void updateButtons()
{
getButton(IDialogConstants.OK_ID).setText("Send");
getButton(IDialogConstants.CANCEL_ID).setText("Skip");
}
After you add this code, your dialog should be similar to the following figure.
Dialog with Custom Labels for the Default Buttons
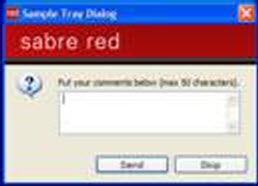
(Optional) Modifying the Behavior of the Default Buttons
-
To handle the selection of the OK and Cancel buttons by end-users, overwrite the okPressed() and cancelPressed() methods. Both the super.okPressed() and super.cancelPressed() methods call an implementation of okPressed() and cancelPressed() from their parent class MySabreTrayDialog in order to use the pre-defined behavior that occurs after the OK and Cancel buttons are clicked.
/**
* {@inheritDoc}
*/
@Override
protected void okPressed()
{
/* OK button code goes here */
super.okPressed();
}
/**
* {@inheritDoc}
*/
@Override
protected void cancelPressed()
{
/* Cancel button code goes here */
super.cancelPressed();
}
(Optional) Creating a New Button
-
Overwrite the createButtonsForButtonBar() method. This method creates default OK and Cancel buttons. Call the method super.createButtonForButtonBar() to create OK and Cancel buttons.
/**
* {@inheritDoc}
*/
@Override
protected void createButtonsForButtonBar(Composite parent)
{
super.createButtonsForButtonBar(parent);
createButton(parent, HELP_BUTTON_ID, "Help", false);
}
Using Eclipse to Create a New Button ID
A new button requires you to pass the Eclipse Button ID. Be careful about creating the unique ID. You cannot use a Button ID that Eclipse defines. Eclipse assigns the numbers 0
and 1
to IDialogConstants
. Therefore, you are advised to use a numbering scheme that differs from the numbering scheme of Eclipse.
Using Java to Create a New Button ID
The preferred way to add a new button is to define HELP_BUTTON_ID as a Java constant. In the code sample above, the Java constant HELP_BUTTON_ID
is a unique ID. The following example shows a line of Java code that defines the unique Java constant. The identifier is final int.
private static final int HELP_BUTTON_ID = 515;
-
Assign your text to the buttons. Add setText to the updateButtons() method. (Refer to the updateButtons() method in a previous step.) The following example assigns the text Send, Skip, and Help to the buttons.
private static final int HELP_BUTTON_ID = 515;
/**
* {@inheritDoc}
*/
@Override
public void updateButtons()
{
getButton(IDialogConstants.OK_ID).setText("Send");
getButton(IDialogConstants.CANCEL_ID).setText("Skip");
getButton(HELP_BUTTON_ID).setText("Help");
}
-
Provide handling for the selection of the new button. Overwrite the buttonPressed() method. In this example, we call super.buttonPressed() to handle the selection of OK and Cancel correctly. You must use the same Button ID to select the button that you used to create the button. In this case, the ID is the HELP_BUTTON_ID constant. Again, HELP_BUTTON_ID is the Java constant that was defined in a previous step.
The helpPressed() method contains code that handles the selection of the Help button.
/**
* {@inheritDoc}
*/
@Override
protected void buttonPressed(int buttonId)
{
super.buttonPressed(buttonId);
if (buttonId == HELP_BUTTON_ID)
{
helpPressed();
}
}
private void helpPressed()
{
/* Add your Help button code here */
}
After you add this code, your dialog should be similar to the following figure.
Dialog with Custom Labels for the Default Buttons and a New Button
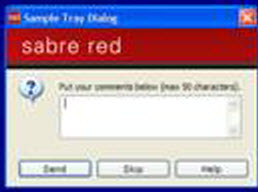
(Optional) Removing a Default Button
-
Overwrite the createButtonsForButtonBar() method. When the line createButton with IDialogConstants.Cancel_ID is omitted, the Cancel button is not created. (Review the code that modifies both default buttons in a previous step.)
/**
* {@inheritDoc}
*/
@Override
protected void createButtonsForButtonBar(Composite parent)
{
createButton(parent, IDialogConstants.OK_ID, IDialogConstants.OK_LABEL, true);
}
The parameter for createButton() establishes the default selected button on the dialog.
-
Overwrite the updateButtons() method. Add setText("Send") to change the value from OK to Send. The following code sample shows the override to updateButtons().
/**
* {@inheritDoc}
*/
@Override
public void updateButtons()
{
getButton(IDialogConstants.OK_ID).setText("Send");
}
-
Save the changes to your Java code.
After you add this code, your dialog should be similar to the following figure. We eliminated the code for the default Cancel button and we changed the label on the default OK button to Send.
Dialog with One Button and a Customized Label
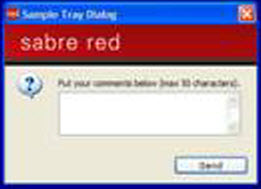