Applications Side Panel
The business purpose of Applications Side Panel is to allow Red Apps to open applications in a dedicated side panel. React is the technology used to create these applications so the Red App itself decides what the application looks like and how it behaves. Unlike other side panels, in this case, we define not the action that will be performed when you select a button in the side panels, but the component that will be rendered.
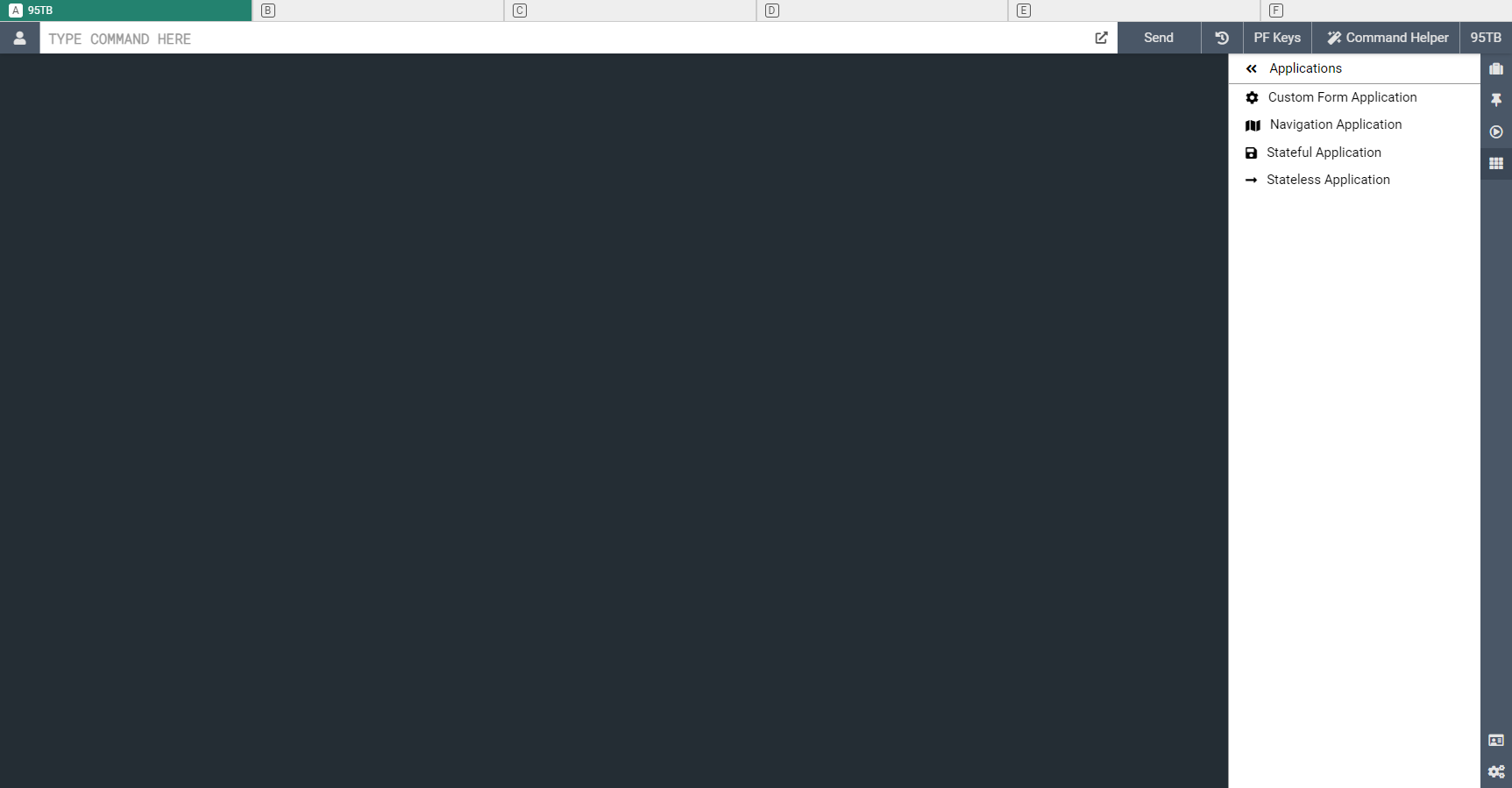
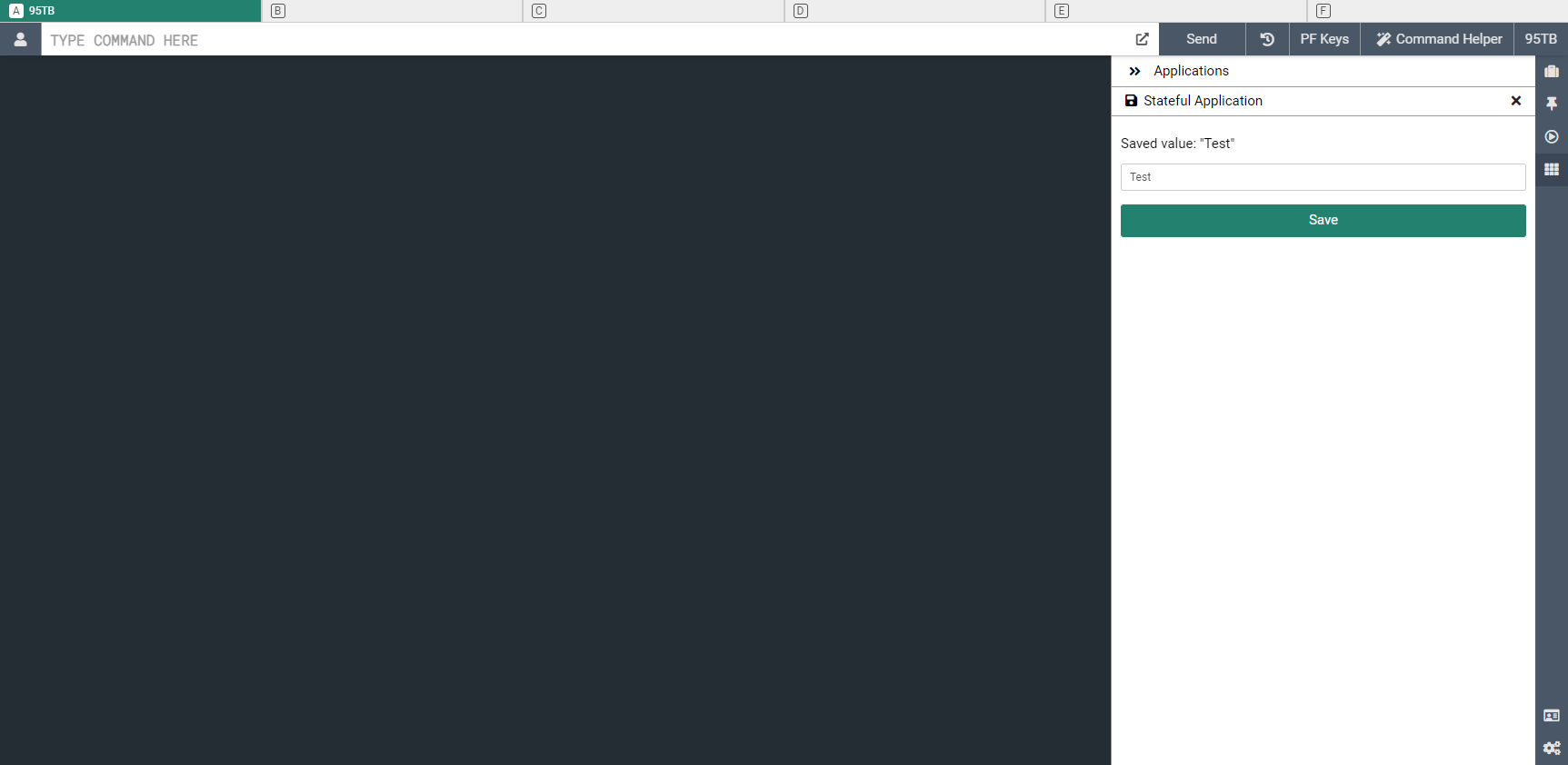
Contributing
Red Apps can contribute to Applications Side Panel via ExtensionPointService. The first step is to add all required imports:
import {AppsSidePanelConfig} from 'sabre-ngv-sdk-apps-sidepanel/configs/AppsSidePanelConfig';
import {AppsSidePanelButton} from 'sabre-ngv-sdk-apps-sidepanel/configs/AppsSidePanelButton';
import {ExtensionPointService} from 'sabre-ngv-xp/services/ExtensionPointService';
Then Red Apps can contribute under appsSidePanel
key as follows:
// Creating Config
const config: AppsSidePanelConfig = new AppsSidePanelConfig([
new AppsSidePanelButton({
// Application ID
id: 'com-example-web-module-example-app',
// Label
label: 'Stateful Application',
// Button Icon
icon: 'fa-save',
// React Component
component: React.createElement(StatefulComponent),
// Redux Store
store,
// Wide Mode
wideMode: true
})
]);
// Adding Config
getService(ExtensionPointService).addConfig('appsSidePanel', config);
Config Properties
The following properties can be defined for config:
Property | Description |
---|---|
buttons |
List of buttons that will be added with this config. |
Button Properties
The following properties can be defined for button:
Property | Description |
---|---|
id |
ID of the application which must be unique to ensure the correct behavior of the application and the Applications Side Panel. To achieve ID uniqueness, use the Web Module name as a prefix (then the suffix needs to be unique only within the Red App). |
label |
Label of the button visible in the side panel that opens the application or header when given application is opened. |
icon |
Icon rendered next to side panel button label. Expects Font Awesome font class (e.g. "fa-cog"). |
component |
The top level React component of the application. |
store (optional) |
Optional Redux store. If defined, it will be provided to the top level component of the application. If the application requires a state, then store is required. The component state is not long-lasting, it is deleted when the application is closed. |
wideMode (optional) |
Optional property that determines whether the application opens in wide (true) or narrow (false) mode by default. If not provided, the application will open in the current mode, or in the mode passed to the |
Opening Application Programmatically
Applications in the Applications Side Panel can be opened programmatically. It is possible to open a specified application or list of all applications in specified width mode (wide or narrow). This can be achieved using IAppsSidePanelService.
IAppsSidePanelService
In order to obtain AppsSidePanelService and use it, you need to import it, e.g.:
import {IAppsSidePanelService} from 'sabre-ngv-sdk-apps-sidepanel/services/IAppsSidePanelService';
Then obtain the service inside your source as below:
const appsSidePanelService: IAppsSidePanelService = getService(IAppsSidePanelService);
Applications can be opened or closed using openApp
method:
appsSidePanelService.openApp('com-example-web-module-example-app', true);
Open App Method Arguments
This method which takes two arguments:
-
id
-
wideMode (optional)
ID
ID of the application to be opened. If the ID is not recognized, a list of all applications will open. If any application was open at that moment, it will be closed.
Wide Mode
Specifies if application should be opened in wide (true) or narrow (false) mode. If this argument is not provided, the configured width mode will be used or the current mode will be left if not configured.